Vector Maps for Android Apps
Advanced User Setup
Using MapLibre SDK for Native apps
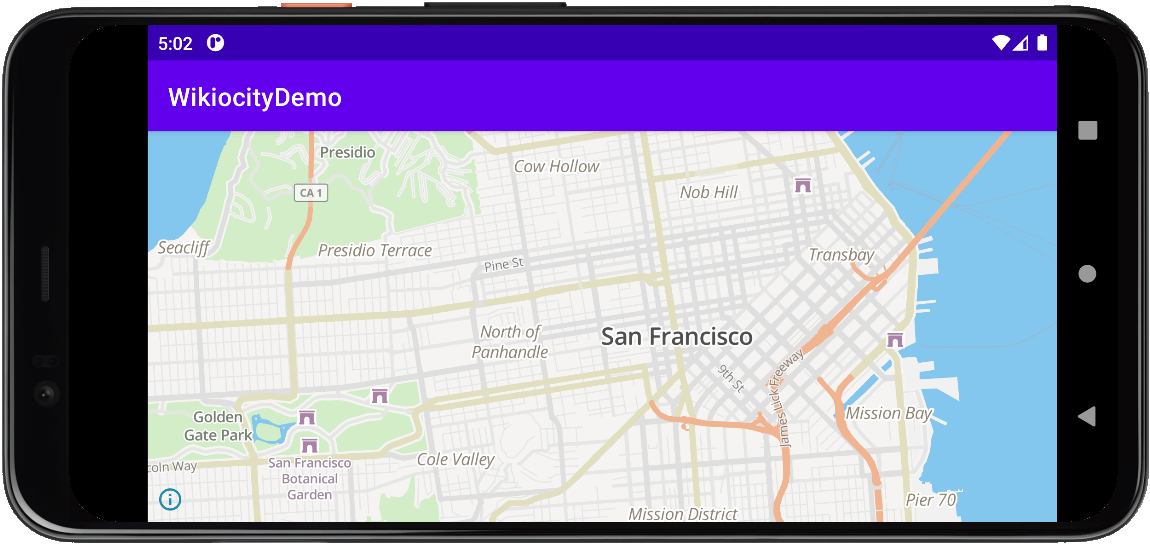
MapLibre SDK
MapLibre is an open source project that was forked from Mapbox GL SDK. It is our preferred mapping program for interactive maps due to its modern design and ease of use.
Link | Description |
---|---|
Homepage | The Projects home for Native SDK |
SDK Reference | Information, examples, and plugins |
Github | Source code |
Use the code below for a quick and easy setup.
New Project Setup
This project will setup a basic interactive map using the MapLibre SDK. Start a new project with the settings below.
Item | Description |
---|---|
Language | Kotlin |
Minimum SDK | API 14 or newer |
Add Dependencies
Follow the instructions for adding dependencies to your project.
Instructions |
---|
From the top drop-down, select File -> Project Structure |
n the Project Structure window, choose Dependencies, then <All Modules>. |
Click + Add Dependency from the <All Modules> column, and then choose Library Dependency from the drop-down. |
Enter org.maplibre.gl:android-sdk: and click Search. Choose the latest version and click OK |
Note: The latest current Android version can be found on the MapLibre SDK release page. |
Repeat clicking + Add Dependency from the <All Modules> column, and then choose Library Dependency from the drop-down. |
Enter com.squareup.okhttp3:okhttp: and click Search. Choose the latest version and click OK |
Your Project build.gradle should now contain the "mavenCentral()" repository. |
Your Module build.gradle should now contain the "org.maplibre.gl:android-sdk:x.x.x" and "com.squareup.okhttp3:okhttp:x.x.x" implementation. |
Setup Layout
Instructions |
---|
From the Project File Navigation Panel, navigate to app -> res -> layout and open activity_{type}.xml |
Note: The activity xml file name is based on the type selected when starting the project. ie - Basic, Empty, or Fullscreen Activity. |
Using Code view, Add the MapView code below within the main Layout, and replace any existing TextViews or other Views. |
<com.mapbox.mapboxsdk.maps.MapView
android:id="@+id/mapView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
Attribution
The next Activity section will already include the links below.
Our maps are built with the help of thousands of public and private sources and open projects. Allowing it's display satisfies their required attributions.
© <a href="https://www.slpy.com">Slpy</a>
© <a href="https://www.openstreetmap.org/copyright">OpenStreetMap</a> contributors
Setup Activity
Instructions |
---|
From the Project File Navigation Panel, navigate to app -> java -> com.examply.{projectname} and open {type}Activity |
Note: The activity file name is based on the type selected when starting the project. ie - Basic, Empty, or Fullscreen Activity. |
Click ... next to import, and add the import code below the other import lines. |
Within the main AppCompatActivity() class, modify or replace the onCreate function with the onCreate code below. |
Note: Change the Api Key, center, zoom, language, and R.layout.activity_{type} to match your needs. | Within the main AppCompatActivity() class, add override code for App events. |
Import Section
import com.mapbox.mapboxsdk.Mapbox
import com.mapbox.mapboxsdk.WellKnownTileServer
import com.mapbox.mapboxsdk.camera.CameraPosition
import com.mapbox.mapboxsdk.maps.MapboxMapOptions
import com.mapbox.mapboxsdk.geometry.LatLng
import com.mapbox.mapboxsdk.maps.MapView
onCreate
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
val apiKey = "Your Api Key"
var center = LatLng(37.78, -122.43)
var startZoom = 12.0
val mapLanguage = "en"
val mapStyle = "slpy-mgl-style.json"
val mapStyleUrl = "https://api.slpy.com/style/${mapStyle}";
Mapbox.getInstance(this, null, WellKnownTileServer.MapLibre)
setContentView(R.layout.activity_{type})
val mglOptions = MapboxMapOptions.createFromAttributes(this, null)
.camera(
CameraPosition.Builder()
.target(center)
.zoom(startZoom)
.build()
).logoEnabled(false)
.attributionMargins(intArrayOf(20, 0, 0, 20))
var mapView = MapView(this, mglOptions)
mapView?.onCreate(savedInstanceState)
mapView?.getMapAsync { map ->
map.setStyle(mapStyleUrl) {
map.uiSettings.setDisableRotateWhenScaling(true)
}
}
setContentView(mapView);
}
overrides
override fun onStart() {
super.onStart()
mapView?.onStart()
}
override fun onResume() {
super.onResume()
mapView?.onResume()
}
override fun onPause() {
super.onPause()
mapView?.onPause()
}
override fun onStop() {
super.onStop()
mapView?.onStop()
}
override fun onSaveInstanceState(outState: Bundle) {
super.onSaveInstanceState(outState)
mapView?.onSaveInstanceState(outState)
}
override fun onLowMemory() {
super.onLowMemory()
mapView?.onLowMemory()
}
override fun onDestroy() {
super.onDestroy()
mapView?.onDestroy()
}
Build and Run
That's it. You should have a working interactive map.
Next Steps
Customize and add features to your new map
Settings & Features
- Common settings and Language Support.
- Content Filtering
- Satellite, Street Level, and other features.
- Compatibility for older browsers.
Map Design
- Customize your maps look
- Night Mode, Greyscale.
- Get the Slpy Style source code.
Search & Geocoding
- Add a search bar to your map.
- Translate addresses to coordinates
- Search for points of interest.