Vector Maps for Apple iOS Apps
Advanced User Setup
Using MapLibre SDK for Native apps
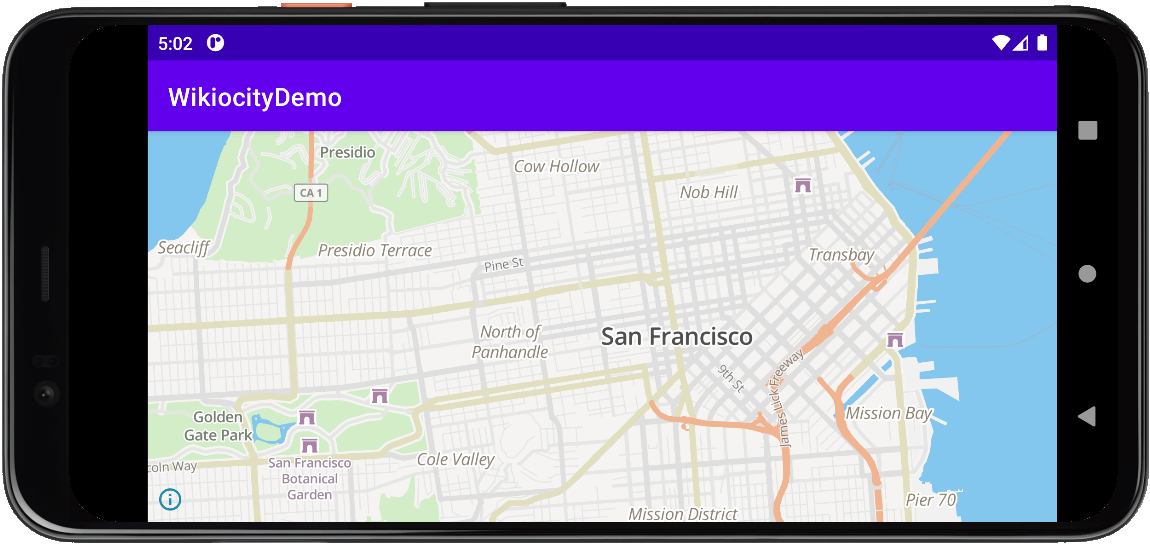
MapLibre SDK
MapLibre is an open source project that was forked from Mapbox GL SDK. It is our preferred mapping program for interactive maps due to its modern design and ease of use.
Link | Description |
---|---|
Homepage | The Projects home for Native SDK |
SDK Reference | Information, examples, and plugins |
Github | Source code |
Use the code below for a quick and easy setup.
New Project Setup
This project will setup a basic interactive map using the MapLibre SDK. Start a new Xcode Project (UIKit) with the settings below.
Item | Description |
---|---|
Platform | iOS App |
Interface | Storyboard |
Language | Swift |
Add Dependencies
Follow the instructions for adding dependencies to your project.
Instructions |
---|
From the top drop-down, select File -> Add Packages |
In the Package Dependency window, search for https://github.com/maplibre/maplibre-gl-native-distribution, then press enter. |
You should see maplibre-gl-native-distribution in the results. Select, then click Add Package |
In the Choose Package Products window, checkmark Mapbox, then click Add Package |
MapLibre GL Native x.x.x should now show under Package Dependencies in the Project Navigation panel. |
Attribution
The next ViewController section will already include the links below.
Our maps are built with the help of thousands of public and private sources and open projects. Allowing it's display satisfies their required attributions.
© <a href="https://www.slpy.com">Slpy</a>
© <a href="https://www.openstreetmap.org/copyright">OpenStreetMap</a> contributors
Setup ViewController
Instructions |
---|
From the Project Navigation Panel, navigate to {App Name} -> {App Name} -> ViewController and open ViewController.swift |
>Note: The App Name is based on the name given when starting the project. |
Find the import code at the top, and add the import section code below the other import lines. |
Within the main ViewController class, modify or replace the viewDidLoad function with the viewDidLoad code below. |
Note: Change the Api Key, center, zoom, and language to match your needs. |
Import Section
import Mapbox
viewDidLoad
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
let apiKey = "Your Api Key"
let center = CLLocationCoordinate2D(latitude: 37.78, longitude: -122.43)
let startZoom = 12.0
let mapLanguage = "en"
let mapStyle = "slpy-mgl-style.json"
let mapStyleUrl = URL(string: "https://api.slpy.com/style/\(mapStyle)?key=\(apiKey)&lang=\(mapLanguage")
let mapView = MGLMapView(frame: view.bounds, styleURL: mapStyleUrl)
mapView.autoresizingMask = [.flexibleWidth, .flexibleHeight]
mapView.setCenter(center, zoomLevel: startZoom, direction: 0, animated: false)
mapView.logoView.isHidden = true
mapView.delegate = self
self.view.addSubview(mapView)
}
Build and Run
That's it. You should have a working interactive map.
Next Steps
Customize and add features to your new map
Settings & Features
- Common settings and Language Support.
- Content Filtering
- Satellite, Street Level, and other features.
- Compatibility for older browsers.
Map Design
- Customize your maps look
- Night Mode, Greyscale.
- Get the Slpy Style source code.
Search & Geocoding
- Add a search bar to your map.
- Translate addresses to coordinates
- Search for points of interest.